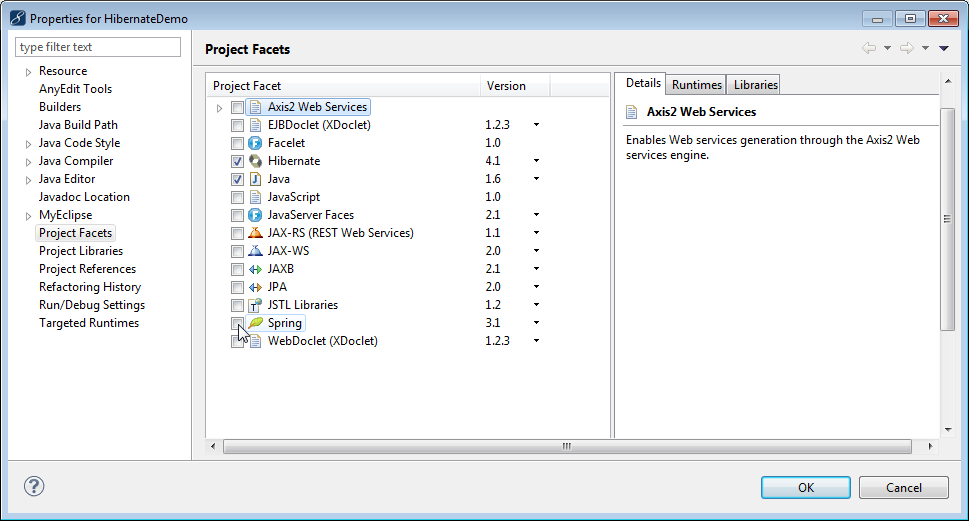
JPA Development
JPA helps control the mapping of POJOs in database tables. It’s a type of persistence specification that is now part of the Java EE 5 spec. Here you will learn to:
- Configure a JPA project
- Reverse engineer entities and DAOS from database
- Use advanced entity editing tools and Spring-JPA integration
This feature is available in MyEclipse.
1. JPA Project Configuration
JPA is a Hibernate-like persistence specification that became part of the Java EE 5 spec. JPA uses Java 5 annotations to control how plain Java classes (POJOs) get mapped to database tables.
You can create a JPA-enabled project by creating any of the supported types of base projects, like a Java or Web project, and then adding JPA facets to that project from the MyEclipse menu. To add JPA facets, right-click the project, and select MyEclipse>Project Facets>Install JPA Facet from the menu. You can also add multiple facets to a project simultaneously by opening the Project Facets propety of the project.
Adding facet via project properties
Persistence Providers
MyEclipse provides support for the OpenJPA, Hibernate, and EclipseLink JPA providers.
Adding JPA facets
Database Configuration
Associate your project with a database and schema for design-time tool support. The project in the image below is being associated with the database driver for the local MyEclipse Derby database.
Configuring the datasource
Instead of relying only on libraries shipped with MyEclipse, you can download libraries to include in your project. Click the Download icon to add a user library.
Downloading a user library
You can change a JPA project’s database driver association at any time using the Java Persistence properties page. To open this page, right-click the project, and select Properties from the menu. Expand MyEclipse>Project Facets, and select Java Persistence.
Java Persistence properties page
2. Reverse Engineering Entities and DAOs from Database
Right-click a JPA project, and select Generate Entities & DAOs from the menu. You are given an option to use either the MyEclipse reverse-engineering tools or the DALI entity generator. Making a select launches the respective JPA Reverse Engineering wizard.
Entity generation from JPA project
You can also generate entities by right-clicking a table in the DB Browser view.
Entity generation from DB table
The reverse engineering process is fully customizable. Using the JPA Reverse Engineering wizard you can choose the artifacts to generate and the database tables from which the artifacts are based.
Customize reverse engineering
MyEclipse can also generate DAOs with result pagination support for `findBy<property>` queries. The generated result pagination API provides DAO clients fine-grained programmatic control to position to a specific row number of a result set and fetch n entities.
Following are several sample snippets of the code generated by the Reverse Engineering processor.
Sample snippets
3. Advanced Entity Editing Tools
MyEclipse Java Persistence Perspective
The MyEclipse Java Persistence perspective provides an optimal editor and view layout for JPA-oriented tasks.
MyEclipse Persistence perspective
JPA Details View
The JPA Details view makes it easy to edit entity annotations.
JPA Details view
JPA Diagram Editor
The JPA diagram editor allows you to easily create new entities or edit entities in your existing JPA projects.
JPA diagram editor
JPA Annotation Table and Column Content Assist
Table content assist
JPA annotation column content assist
JPA Entity Validation
Errors in your mapping are detected and displayed in the editor and Problems view.
JPA validation errors shown in Java editor
The JPA Entity Validator can be enabled or disabled at the project level.
JPA Validation preferences
4. Advanced Spring-JPA Integration
Spring 2 Support
When adding JPA facets to a project that already has Spring facets or vice versa, you can choose advanced Spring-JPA support. This level of support enables JPA tools to work with your project’s Spring artifacts. The following image shows the Spring-JPA project configuration wizard.
Adding Spring capabilities
Select Spring-JPA support, your primary bean configuration file, and customize bean Ids and transaction support.
Configure Spring-JPA support
Reverse Engineering Entities and Spring DAOs from Database
For projects that are configured to support advanced Spring-JPA capabilities, in addition to generating Entity classes from a database schema, Spring-compatible DAOs can be generated. During the reverse-engineering process, the Spring application context file is updated with generated bean entries for each DOA class.
Generating Spring DAOs
Generated Spring application context file
Generated Spring DAO extends from Spring’s JpaDaoSupport