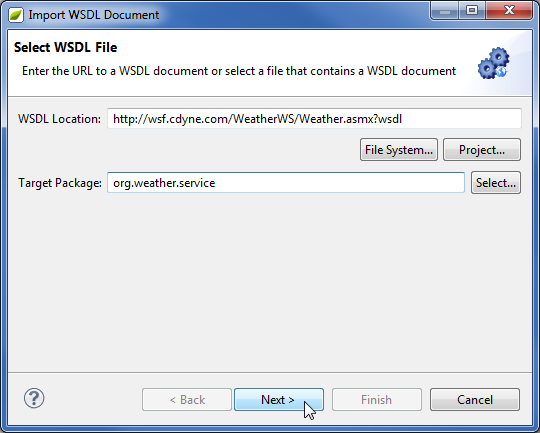
Importing a SOAP Web Service into a Spring Application
There is a variety of functions to facilitate SOAP web services development. This tutorial is specifically focused on consuming a third party web service from within a Spring application. This is accomplished by using the Import Web Services wizard. In this tutorial, you will learn how to:
- Import a WSDL into an existing project
- View the WSDL in the editor
- Test a web service
This feature is available in MyEclipse
1. Import a WSDL
This tutorial uses the free CDYNE Weather web service, which provides weather information in the United States by zip code. Please read the notes section on the CDYNE Wiki site for information regarding this web service. The WSDL for the CDYNE Weather web service is http://wsf.cdyne.com/WeatherWS/Weather.asmx?wsdl. If this does not work, please consult the CDYNE Wiki site.
- Create a scaffolded CustomersApp project.
- Right-click CustomersApp, and select Spring Tools>Import WSDL
This menu option was updated in MyEclipse 2017. For prior versions, click here. .
The Import Web Services wizard helps consume external SOAP web services from within applications by generating the Java classes needed for interacting with the web services.
The Import Web Services wizard also helps you implement a service contract (referred to as Contract-First development) by stubbing out a concrete implementation of the service from a WSDL (SOAP web service contract). - Enter the following WSDL URL into the WSDL Location field: http://wsf.cdyne.com/WeatherWS/Weather.asmx?wsdl, and type org.weather.service in the Target Package field. Click Next.
Specifying the WSDL URL - Accept the default project configuration options, and click Next.
Configuration options - Accept the default JAX-WS settings, and click Next.
Setting JAX-WS options - Accept the default Spring Capabilities settings, and click Next.
Setting JAX-WS options - Accept the default libraries, and click Next.
Project libraries - Click Finish on the Summary page. All the necessary source code for using the web service is generated.
Summary page
2. Review Generated Code
By default the Import Web Service wizard generates source code to the generated folder. Since the folder doesn’t exist, the wizard creates the folder and sets it up as an Eclipse source folder. All artifacts created reflect the web service’s definition in the WSDL.
Generated Java files from the WSDL
The com.dyne.ws.weatherws package contains the source code generated for the WSDL entities. The package name is based on the target namespace specified in the WSDL.
The org.weather.service package contains the Spring service interface, Spring service implementation (Spring @Service), and Junit test. This source code is used only if you are implementing the service based on the contract (Contract-First Development).
The org.weather.service.jaxws package contains the service endpoint interface and service client. The service endpoint interface should have a Java method for web service operation.
2.1 Viewing the WSDL
- Open the resources/wsdls/com/cdyne/ws/wsdl/asmx/weather/weatherws/ folder.
- Right-click weatherws.wsdl, and select Open With>MyEclipse WSDL Editor. The WSDL editor displays a graphical view of the service, including operations, complex types, and relationships.
WSDL editor
3. Test the Web Service
This section shows you how to use the generated source code to test the web service. This is accomplished by adding a call to the web service from a preexisting method, specifically the `loadCustomers()` method in the CustomerServiceImpl class.
- Add the following import statements to CustomerServiceImpl.java (generated/org/customerapp/service).
import org.weather.service.jaxws.IWeatherSoapEndpoint; import org.weather.service.jaxws.WeatherSoapClient; import com.cdyne.ws.weatherws.ForecastReturn;
- Update the `loadCustomers()` method in CustomerServiceImpl.java (generated/org/customerapp/service) with the following lines of code that don’t already exist. This is just a basic example. The web service has other methods that are available.
public Set<Customer> loadCustomers() { WeatherSoapClient wsClient = new WeatherSoapClient(); IWeatherSoapEndpoint service = wsClient.getService(); ForecastReturn fr = service.GetCityForecastByZIP("33710"); System.out.println("Acquired weather for "+fr.getCity()+" "+fr.getState()); return customerDAO.findAllCustomers(); }
- Deploy the CustomersApp project, and browse to http://localhost:8080/CustomersApp/indexCustomer. This URL calls the service method modified in the previous step. The Java console should indicate that the service was called.